DOM Events
Making things interactive
Events are everywhere
- Clicking on a button
- Hovering over a link
- Dragging and Dropping
- Pressing the Enter key
The Process
We select an element and then add an event listener
"Listen for a click on this <button>"
"Listen for a hover event on the <h1>"
"Listen for a keypress event on text input"
The Syntax
To add a listener, we use a method called addEventListener
var button = document.querySelector("button");
button.addEventListener("click", function() {
console.log("SOMEONE CLICKED THE BUTTON!");
});
element.addEventListener(type, functionToCall);
An Example
Let's display a message when a button is clicked
var button = document.querySelector("button");
var paragraph = document.querySelector("p");
//SETUP CLICK LISTENER
button.addEventListener("click", function() {
paragraph.textContent = "Someone Clicked the Button!";
});
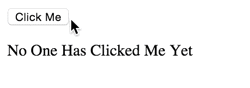
<button>Click Me</button>
<p>No One Has Clicked Me Yet</p>
An Example
We could also rewrite it using a named function
var button = document.querySelector("button");
var paragraph = document.querySelector("p");
button.addEventListener("click", changeText);
function changeText() {
paragraph.textContent = "Someone Clicked the Button!";
}
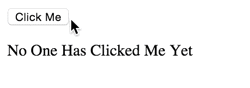
So Many Events!
MDN lists over 300 different events! Here are some of the more common ones:
- click
- mouseover
- dblclick
- keypress
- drag
Another Example
var paragraph = document.querySelector("p");
//SETUP MOUSE OVER LISTENER
paragraph.addEventListener("mouseover", function() {
paragraph.textContent = "Stop hovering over me!";
});
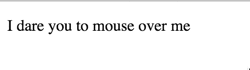
<p>I dare you to mouse over me</p>
Let's try a quick example using mouseOver
Adding mouseout
var paragraph = document.querySelector("p");
//SETUP MOUSE OVER LISTENER
paragraph.addEventListener("mouseover", function() {
paragraph.textContent = "Stop hovering over me!";
});
//SETUP MOUSE OUT LISTENER
paragraph.addEventListener("mouseout", function() {
paragraph.textContent = "Phew, thank you for leaving me alone";
});
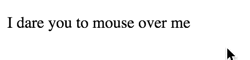
Let's use mouseout so that our message changes back when the user is done hovering
A Minor Change
var paragraph = document.querySelector("p");
//SETUP MOUSE OVER LISTENER
paragraph.addEventListener("mouseover", function() {
this.textContent = "Stop hovering over me!";
});
//SETUP MOUSE OUT LISTENER
paragraph.addEventListener("mouseout", function() {
this.textContent = "Phew, thank you for leaving me alone";
});
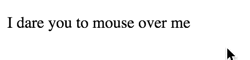
We can DRY up our code with one small change:
Printer Friendly - DOM Events
By Colt Steele
Printer Friendly - DOM Events
- 1,934