DOM Selectors
Document
It all starts with the document, the root node
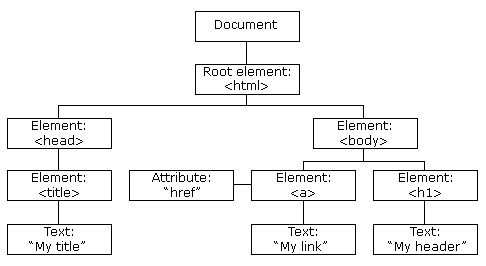
Exercise
Open up the JS console and try these 4 lines:
document.URL;
document.head;
document.body;
document.links;
Methods
The document comes with a bunch of methods for selecting elements. We're going to learn about the following 5:
- document.getElementById()
- document.getElementsByClassName()
- document.getElementsByTagName()
- document.querySelector()
- document.querySelectorAll()
getElementById
var tag = document.getElementById("highlight");
Takes a string argument and returns the one element with a matching ID
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
getElementsByClassName
var tags = document.getElementsByClassName("bolded");
console.log(tags[0]);
Takes a string argument and returns a list of elements that have a matching class
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
getElementsByTagName
var tags = document.getElementsByTagName("li");
console.log(tags[0]);
Returns a list of all elements of a given tag name, like <li> or <h1>
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
getElementsByTagName
var tags = document.getElementsByTagName("h1");
console.log(tags[0]);
Returns a list of all elements of a given tag name, like <li> or <h1>
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
querySelector
//select by ID
var tag = document.querySelector("#highlight");
Returns the first element that matches a given CSS-style selector
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
querySelector
//select by Class
var tag = document.querySelector(".bolded");
Returns the first element that matches a given CSS-style selector
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
querySelector
//select by tag
var tag = document.querySelector("h1");
Returns the first element that matches a given CSS-style selector
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
querySelectorAll
//select by tag
var tags = document.querySelectorAll("h1");
Returns a list of elements that matches a given CSS-style selector
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
querySelectorAll
//select by class
var tags = document.querySelectorAll(".bolded");
Returns a list of elements that matches a given CSS-style selector
<body>
<h1>Hello</h1>
<h1>Goodbye</h1>
<ul>
<li id="highlight">List Item 1</li>
<li class="bolded">List Item 2</li>
<li class="bolded">List Item 3</li>
</ul>
</body>
Exercise
<!DOCTYPE html>
<html>
<head>
<title>My title</title>
</head>
<body>
<h1>I am an h1!</h1>
<p id="first" class="special">Hello</p>
<p class="special">Goodbye</p>
<p>Hi Again</p>
<p id="last">Goodbye Again</p>
</body>
</html>
Come up with 4 different ways to select the first <p> tag
DOM Selectors
By Colt Steele
DOM Selectors
- 21,146